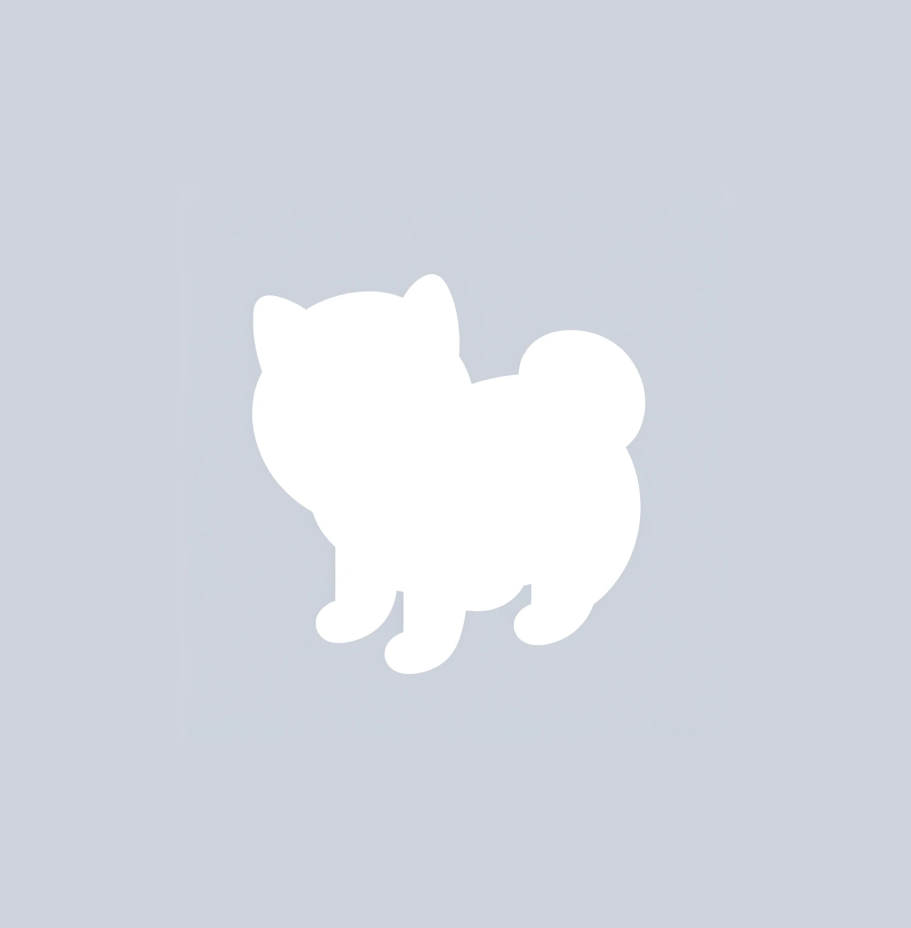
2024/9/21
Closure javascript閉包 Closure
什麼是閉包 (Closure)?
當內部 (inner) 函式被回傳後,除了自己本身的程式碼外,也可以 穿越 取得了內部函式「當時環境」的變數值,記住了執行當時的環境,這就是「閉包」。
參考 Kuro 大這篇文章說明:重新認識 JavaScript: Day 19 閉包 Closure
舉個簡單的例子:
一個計數器的例子,每次調用內部函式時,count 增加,並返回當前的 count 值。
function createCounter() {
let count = 0; // 外部函式中的變數
return function () {
// 內部函式,形成閉包
count += 1; // 每次調用內部函式時,count 增加
return count; // 返回當前的 count 值
};
}
const counter = createCounter(); // 建立閉包
console.log(counter()); // 1
console.log(counter()); // 2
console.log(counter()); // 3
但是我今天建立不同的變數去儲存閉包,發現每個變數都是獨立的,不會互相影響。
function createCounter() {
let count = 0; // 外部函式中的變數
return function () {
// 內部函式,形成閉包
count += 1; // 每次調用內部函式時,count 增加
return count; // 返回當前的 count 值
};
}
const counter1 = createCounter(); // 建立閉包1
const counter2 = createCounter(); // 建立閉包2
const counter3 = createCounter(); // 建立閉包3
//測試閉包1
console.log(counter1()); // 1
console.log(counter1()); // 2
console.log(counter1()); // 3
//測試閉包2
console.log(counter2()); // 1
console.log(counter2()); // 2
//測試閉包3
console.log(counter3()); // 1
所以閉包是可以取得內部函式,並取得當前變數,並記住當時的上下文環境。且每個閉包是獨立的,不會互相影響。
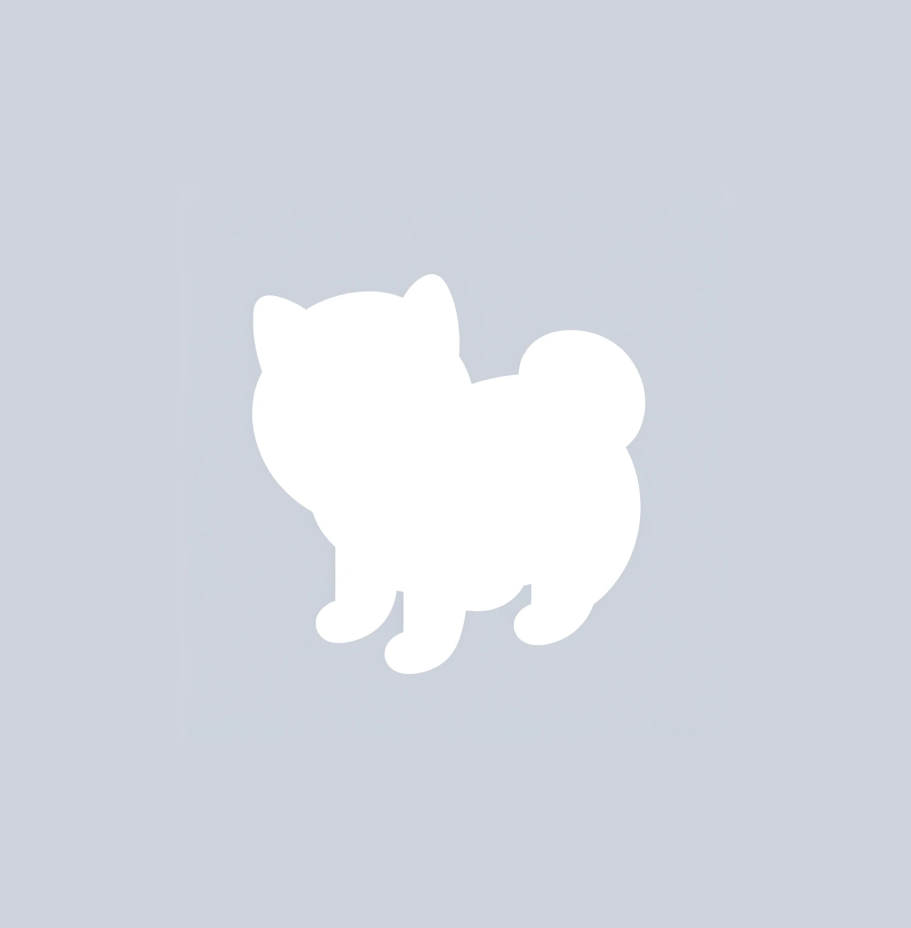
2024/9/5
javascript axios仿axios套件實做
嘗試模仿axios試做一個簡單的get請求
const url="https://randomuser.me/api/"
const axios ={
get:function(url){
return new Promise((reslove,reject)=>{
const xhr = new XMLHttpRequest();
xhr.open('GET',url);
xhr.onload = ()=>reslove(xhr.responseText);
xhr.onerror=()=>reject(xhr.statusText);
xhr.send();
})
}
}
axios.get(url).then(data=>console.log(data)).catch(err=>console.log(err));
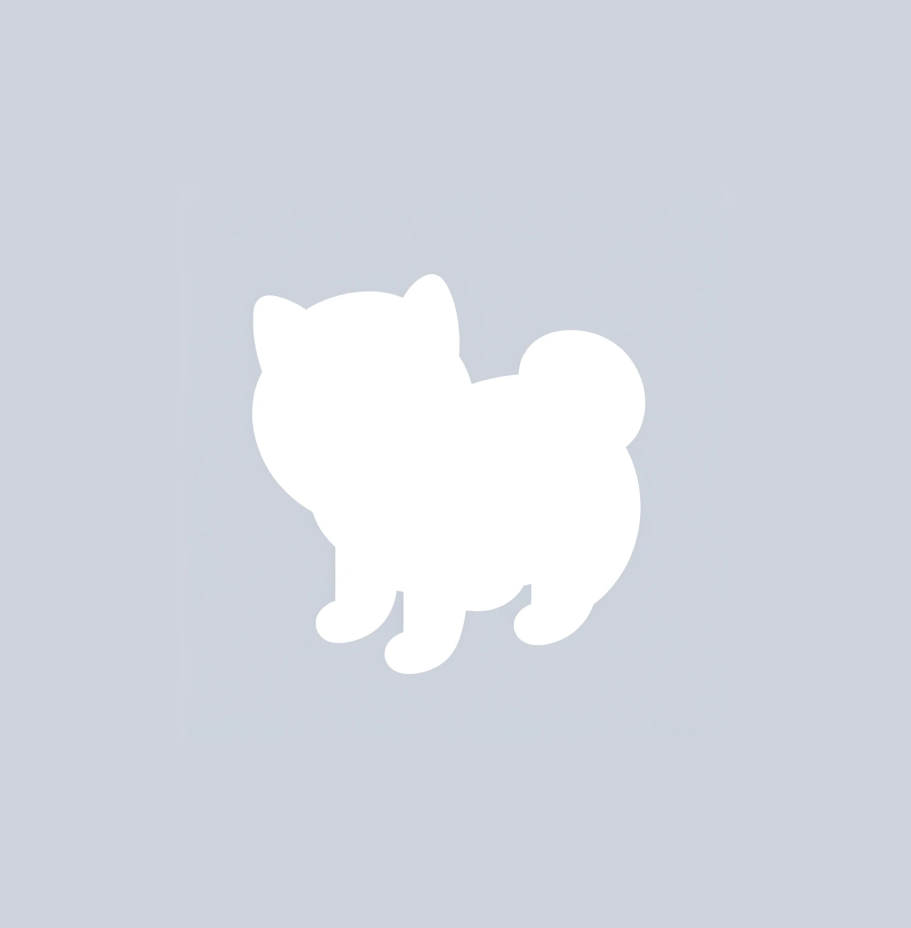
2024/6/11
React HooksuseRef 和 forwardRef
為什麼要使用 useRef
和 forwardRef
?
useRef
- 可以維持元件的狀態,並且不會觸發重新渲染。
- 可以用來控制DOM元素,例如:focus、scroll、play、pause等。
程式碼範例如下:
function MyComponent() {
const inputRef = useRef(null);
useEffect(() => {
inputRef.current.focus(); // 自動聚焦
}, []);
return <input ref={inputRef} />;
}
forwardRef
- 可以將ref傳遞到子元件。
- 如果不使用
forwardRef
,React會報錯
程式碼範例如下:
// 定義一個 FancyButton 組件,使用 forwardRef 將 ref 傳遞下去
const FancyButton = forwardRef((props, ref) => (
<button ref={ref} className="FancyButton">
{props.children}
</button>
));
function App() {
const buttonRef = useRef(null);
const handleClick = () => {
if (buttonRef.current) {
buttonRef.current.focus(); // 使用 ref 來聚焦按鈕
}
};
return (
<div>
<FancyButton ref={buttonRef}>Click me!</FancyButton>
<button onClick={handleClick}>Focus Fancy Button</button>
</div>
);
}
同場加映:useImperativeHandle
目的是限制暴露的方法給父元件來做使用
// 定義一個自定義輸入框組件,使用 forwardRef 傳遞 ref
const CustomInput = forwardRef((props, ref) => {
const inputRef = useRef();
// 使用 useImperativeHandle 來暴露 focus 方法給父組件
useImperativeHandle(ref, () => ({
focus: () => {
inputRef.current.focus();
}
}));
return <input ref={inputRef} {...props} />;
});
function ParentComponent() {
const inputRef = useRef();
const handleClick = () => {
inputRef.current.focus(); // 使用 ref 來聚焦輸入框
};
return (
<div>
<CustomInput ref={inputRef} placeholder="Click the button to focus" />
<button onClick={handleClick}>Focus Input</button>
</div>
);
}
說明:
- 自定義輸入框組件 CustomInput:使用 forwardRef 來接收 ref 並將其傳遞給內部的 input 元素。
- 使用 useImperativeHandle:這允許我們在父組件中自定義暴露的方法(如 focus)。
- 父組件 ParentComponent:使用 useRef 來創建 ref,並通過按鈕點擊觸發輸入框的聚焦。
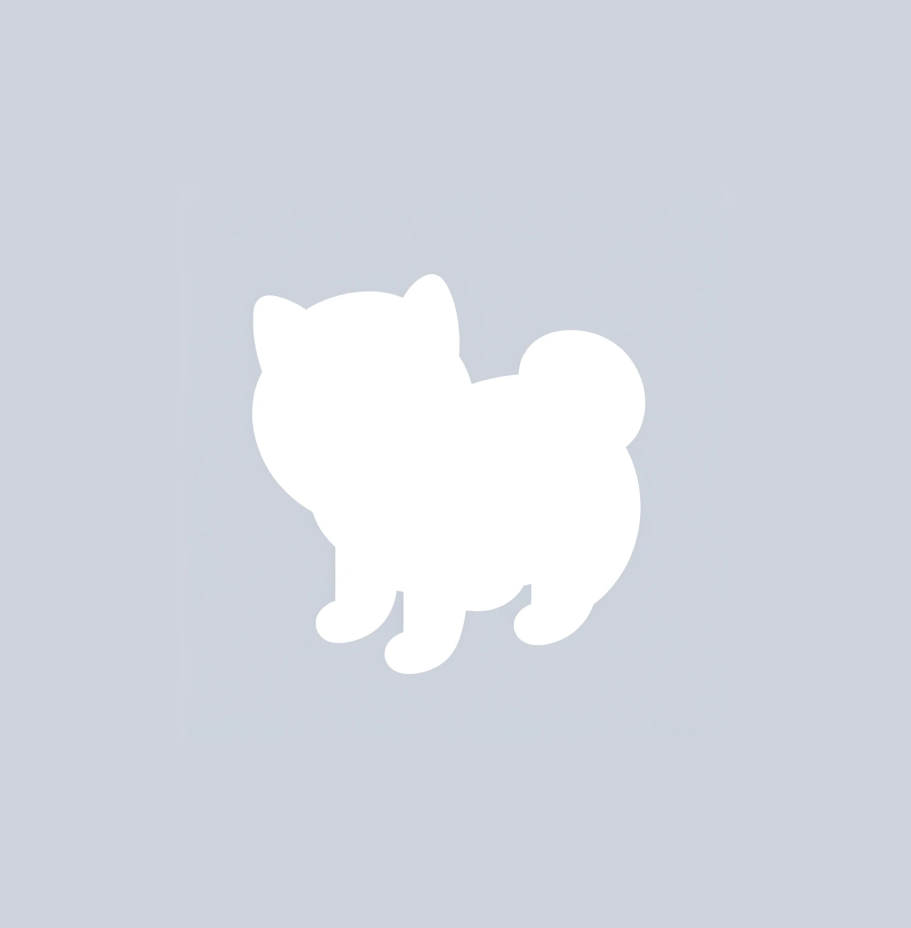
2024/6/6
VS CodeVS Code 常用快捷鍵
這些是我常用的快捷鍵做一下紀錄:
導航
- Cmd + B:切換檔案總管
- Cmd + P:按名稱搜尋檔案
- Cmd + Shift + P:快速進入任何設置
- **Ctrl + `**:切換終端機開關
編輯
- **Cmd +/-**:放大/縮小
- Cmd + Shift + F:在整個專案中搜尋單詞或短語
- Cmd + W:關閉當前檔案
- Cmd + Shift + T:重新打開最近關閉的檔案
- Cmd + D:選擇當前單詞的下一個出現位置以進行多重編輯
- Option + 上/下:移動當前行
- Option + Shift + 上/下:複製當前行
- Cmd + X:剪切當前行
- Cmd + 左/右:移動到行的開始/結束
- Option + 左/右:向左/右移動一個單詞
Finder/Explorer 整合
- 右鍵點擊文件夾:選擇“在文件夾中查找”以僅在特定目錄中搜尋
- 右鍵點擊檔案:選擇“在 Finder 中顯示”(或其作業系統等效選項)以打開檔案位置
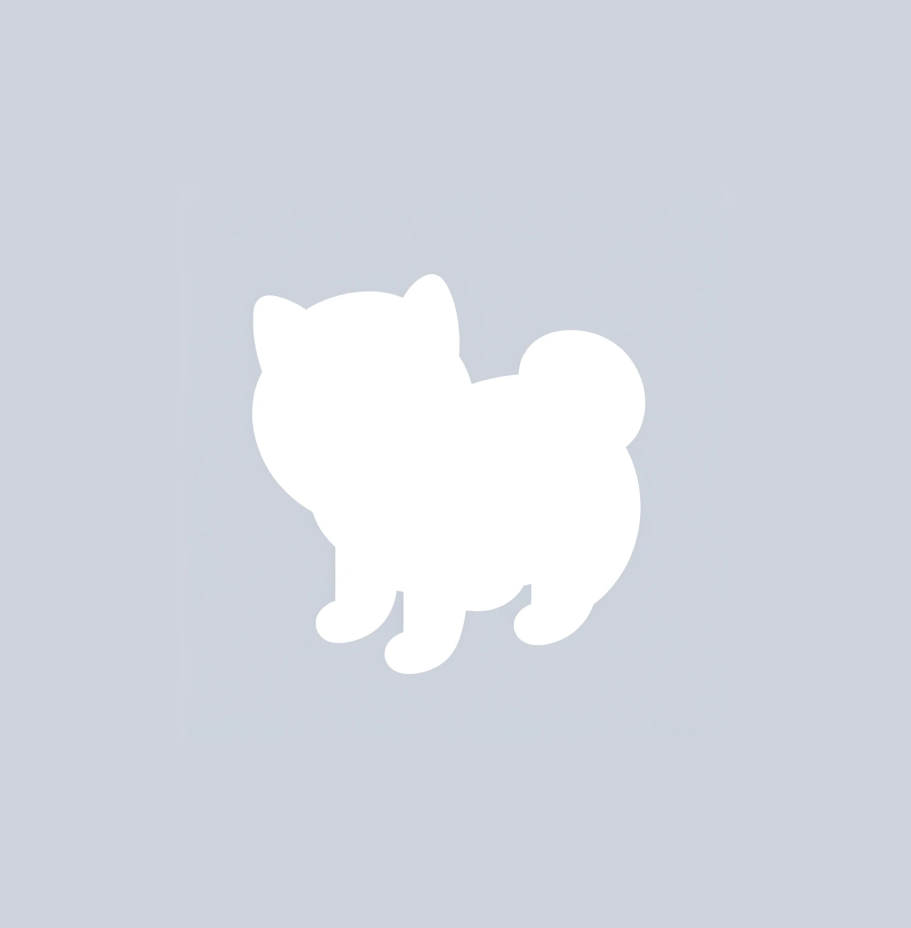
2024/5/30
2024 六角軟體工程師體驗營
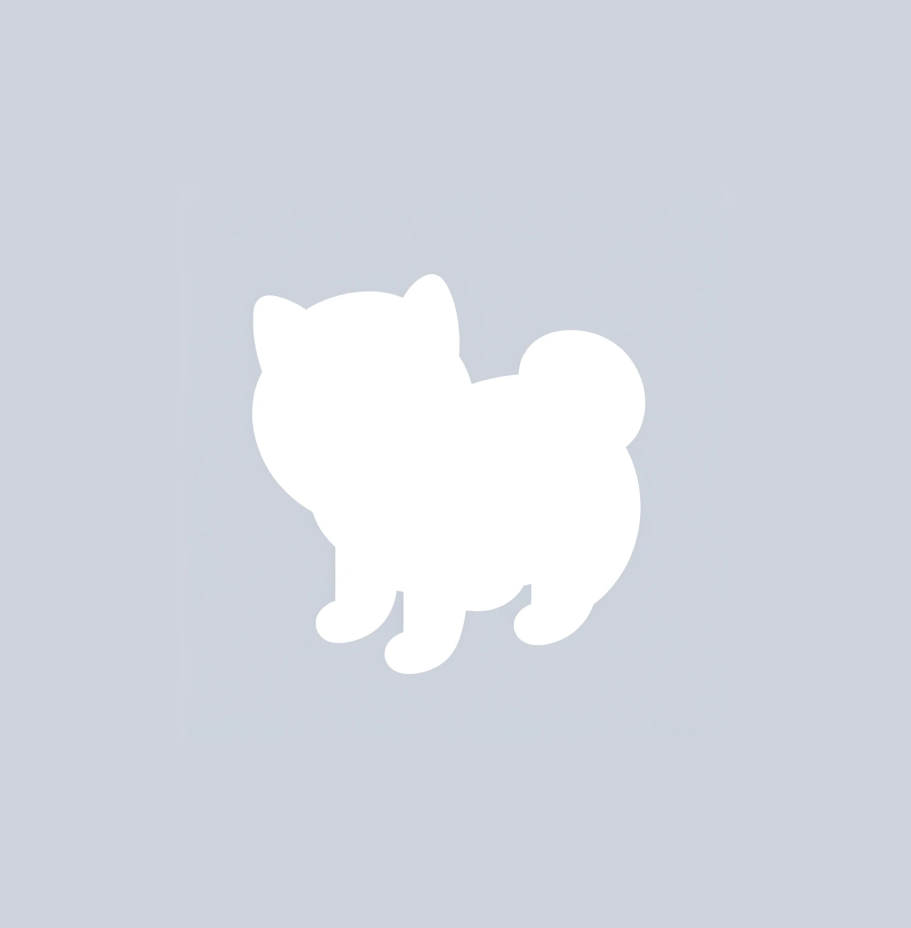
2024/3/6
React HookReact-Hook-useContext
useContext 是一個 React Hook,因為React為單向資料流的特性,所以當我們需要在不同的 component 之間共享資料時,我們通常會透過 props 來傳遞資料,但是當 component 的層級越來越深時,這樣的寫法會變得非常麻煩,這時候 useContext 就可以派上用場,作為一個傳送門useContext可以像下圖中自由的傳遞props。
Ref:dev.react.com
使用方式
1.建立一個 空的context並且導出
常見作法是在建立一個新的檔案中建立一個空的context並且導出,檔名可以隨意取名。
import { createContext } from 'react';
export const MyContext = createContext(null);
2.在最上層的component中使用Provider,並包裹住想要傳遞的元件,傳遞想要傳入的porps
import { MyContext } from './useContext.jsx'
let AAA="132"
<MyContext.Provider value={{AAA}}>
<App />
</MyContext.Provider>
3.可以在porvider包裹住的情況下,使用useContext來取得資料
import { MyContext } from "./useContext.jsx";
const {AAA}=useContext(MyContext)